TensorFlow のライブラリ容量が 1GB 以上もあるので、容易に配布することが出来ない。
なので TensorFlow の計算はサーバー処理でやって、結果だけをクライアントに返せばいいんじゃないかと思い挑戦してみました。
環境
- Windows 10 Home 2004
- Python 3.7.8
- TensorFlow 2.2.0
TensorFlow の計算結果を返すサーバー処理
Python で TensorFlow の計算結果を返すサーバー処理を作成していきます。
TensorFlow 側
ファイル名:TfManager.py
1 2 3 4 5 6 7
| import tensorflow as tf
class TfManager:
@tf.function def MathAdd(self, x, y): return tf.math.add(x, y)
|
引数で来た xの値 と yの値 を足して、結果を返す処理となっています。
HTTP Server 側
ファイル名:http-server.py
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31
| import json from http.server import BaseHTTPRequestHandler, HTTPServer from TfManager import TfManager
class HandleRequests(BaseHTTPRequestHandler):
def do_POST(self): content_len = int(self.headers.get("content-length")) post_body = self.rfile.read(content_len).decode("utf-8") post_json = json.loads(post_body)
x = post_json['x'] y = post_json['y']
tfm = TfManager() result = tfm.MathAdd(x, y) result_val = str(result.numpy())
self.send_response(200) self.send_header('Content-type', 'application/json') self.send_header('Content-Length', len(result_val)) self.end_headers() self.wfile.write(result_val.encode('utf-8')) if __name__ == '__main__': server_address = ('0.0.0.0', 8080) httpd = HTTPServer(server_address, HandleRequests) print('Serving HTTP on localhost port %d ...' % server_address[1]) print('use <Ctrl-(C or break)> to stop') httpd.serve_forever()
|
POST で来た xの値 と yの値 を TfManagerクラス の MathAdd関数 に渡して、その結果をクライアントに返す処理となっています。
動作確認
まず「http-server.py」をダブルクリックし、HTTP Server を立ち上げます。
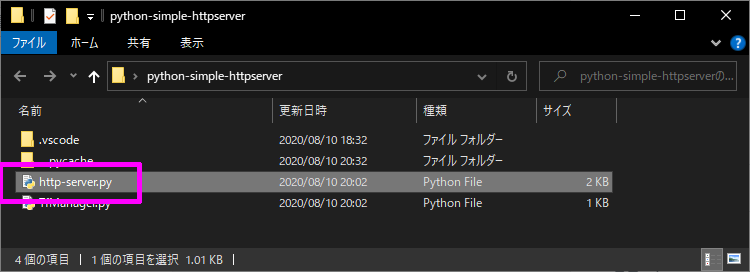
こんな画面が立ち上がれば OK
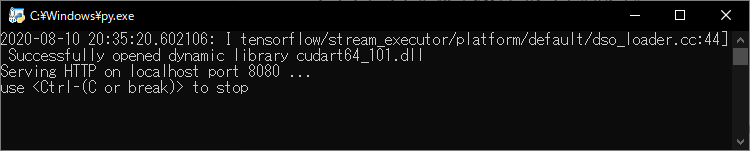
次に、このサーバーに向けて POST をして結果を確認します。
下記コマンドを コマンドプロンプト で実行すると POST する事ができます。
1
| curl -X POST -H "Content-Type: application/json" -d "{""x"": 13, ""y"": 2}" http://localhost:8080/
|
こんな感じに 15 が戻ってこれば成功です。
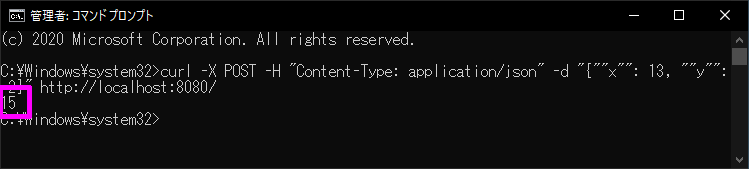